Mastermind Solution - A Fun Coding Challenge

- Published on
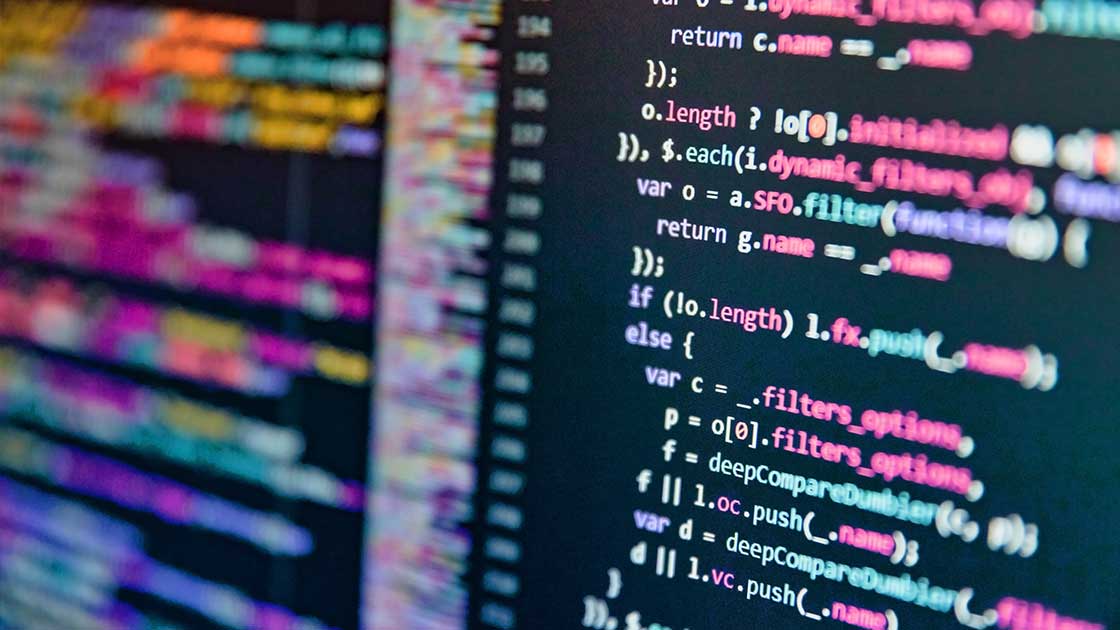
Ready to dive into the world of code-breaking and colorful pegs? You're in the right place! In this article, we'll explore the classic Mastermind game, and provide you with a light-hearted, in-depth guide for implementing it in both Python and JavaScript. Get ready to put your code-cracking skills to the test while having a chuckle or two along the way.
Python Implementation Walkthrough:
- Import the 'random' library
import random
First things first: we need a little bit of randomness in our lives, so let's import the 'random' library to generate a random secret code later on.
- Generate the secret code
def generate_secret_code(colors, code_length):
return [random.choice(colors) for _ in range(code_length)]
Now let's create a magical secret code. This function takes the list of available colors and the desired code length as arguments. It then waves its virtual wand, concocting a secret code by randomly selecting a color for each position.
- Get feedback
def get_feedback(secret_code, guess):
correct_position = sum([1 for i, color in enumerate(guess) if color == secret_code[i]])
correct_color = sum([min(secret_code.count(color), guess.count(color)) for color in set(guess)]) - correct_position
return correct_position, correct_color
This clever little function compares the player's guess to the secret code and returns two top-secret values: the number of colors in the correct position and the number of colors in the code but not in the correct position.
- Main function
def mastermind():
# Initialize variables
colors = ["red", "blue", "green", "yellow", "white", "black"]
code_length = 4
max_attempts = 10
# Generate the secret code
secret_code = generate_secret_code(colors, code_length)
# Print the game instructions
# ...
# Main game loop
for attempt in range(1, max_attempts + 1):
# Get user input
# ...
# Check if the guess is valid
# ...
# Get feedback and check if the user has won
# ...
# Print the result
# ...
Our main function initializes the game variables, generates the secret code, and prints the game instructions. It then jumps into the main game loop, handling user input and checking for a win or loss.
Python Solution
# Python solution for Mastermind game
import random
def generate_secret_code(colors, code_length):
return [random.choice(colors) for _ in range(code_length)]
def get_feedback(secret_code, guess):
correct_position = sum([1 for i, color in enumerate(guess) if color == secret_code[i]])
correct_color = sum([min(secret_code.count(color), guess.count(color)) for color in set(guess)]) - correct_position
return correct_position, correct_color
def mastermind():
colors = ["red", "blue", "green", "yellow", "white", "black"]
code_length = 4
max_attempts = 10
secret_code = generate_secret_code(colors, code_length)
print("Welcome to the Mastermind game!")
print(f"I have generated a secret code of {code_length} colors.")
print(f"Available colors: {', '.join(colors)}")
print(f"You have {max_attempts} attempts to guess the secret code.\n")
for attempt in range(1, max_attempts + 1):
print(f"Attempt {attempt}:")
guess = input("Enter your guess (separate colors by space): ").split()
while len(guess) != code_length or any(color not in colors for color in guess):
print("Invalid input. Please enter exactly 4 colors from the available colors list.")
guess = input("Enter your guess (separate colors by space): ").split()
correct_position, correct_color = get_feedback(secret_code, guess)
if correct_position == code_length:
print("Congratulations, you guessed the secret code!")
break
else:
print(f"Feedback: {correct_position} color(s) in the correct position, {correct_color} color(s) in the code but not in the correct position.\n")
else:
print(f"Sorry, you didn't guess the secret code. It was {', '.join(secret_code)}.")
mastermind()
JavaScript Implementation Walkthrough:
- Generate the secret code
function generateSecretCode(colors, codeLength) {
const secretCode = [];
for (let i = 0; i < codeLength; i++) {
secretCode.push(colors[Math.floor(Math.random() * colors.length)]);
}
return secretCode;
}
Just like its Python cousin, this function generates a random secret code based on the list of available colors and desired code length.
- Get feedback
function getFeedback(secretCode, guess) {
let correctPosition = 0;
let correctColor = 0;
for (let i = 0; i < secretCode.length; i++) {
if (guess[i] === secretCode[i]) {
correctPosition++;
} else if (secretCode.includes(guess[i])) {
correctColor++;
}
}
return [correctPosition, correctColor];
}
This function plays detective, comparing the player's guess to the secret code and returning the number of colors in the correct position and the number of colors in the code but not in the correct position. It's a master of code-cracking!
- Main function
function mastermind() {
// Initialize variables
const colors = ["red", "blue", "green", "yellow", "white", "black"];
const codeLength = 4;
const maxAttempts = 10;
// Generate the secret code
const secretCode = generateSecretCode(colors, codeLength);
// Print the game instructions
// ...
// Main game loop
for (let attempt = 1; attempt <= maxAttempts; attempt++) {
// Get user input
// ...
// Check if the guess is valid
// ...
// Get feedback and check if the user has won
// ...
// Print the result
// ...
}
The JavaScript version of our main function follows a similar approach to the Python implementation, initializing the game variables, generating the secret code, and printing the game instructions. It then hops into the main game loop, managing user input and assessing whether the player has won or lost.
JavaScript Solution
// JavaScript solution for Mastermind game
function generateSecretCode(colors, codeLength) {
const secretCode = [];
for (let i = 0; i < codeLength; i++) {
secretCode.push(colors[Math.floor(Math.random() * colors.length)]);
}
return secretCode;
}
function getFeedback(secretCode, guess) {
let correctPosition = 0;
let correctColor = 0;
for (let i = 0; i < secretCode.length; i++) {
if (guess[i] === secretCode[i]) {
correctPosition++;
} else if (secretCode.includes(guess[i])) {
correctColor++;
}
}
return [correctPosition, correctColor];
}
function mastermind() {
const colors = ["red", "blue", "green", "yellow", "white", "black"];
const codeLength = 4;
const maxAttempts = 10;
const secretCode = generateSecretCode(colors, codeLength);
console.log("Welcome to the Mastermind game!");
console.log(`I have generated a secret code of ${codeLength} colors.`);
console.log(`Available colors: ${colors.join(", ")}`);
console.log(`You have ${maxAttempts} attempts to guess the secret code.\n`);
for (let attempt = 1; attempt <= maxAttempts; attempt++) {
console.log(`Attempt ${attempt}:`);
let guess = prompt("Enter your guess (separate colors by space): ").split(" ");
while (guess.length !== codeLength || guess.some(color => !colors.includes(color))) {
alert("Invalid input. Please enter exactly 4 colors from the available colors list.");
guess = prompt("Enter your guess (separate colors by space): ").split(" ");
}
const [correctPosition, correctColor] = getFeedback(secretCode, guess);
if (correctPosition === codeLength) {
console.log("Congratulations, you guessed the secret code!");
break;
} else {
console.log(`Feedback: ${correctPosition} color(s) in the correct position, ${correctColor} color(s) in the code but not in the correct position.\n`);
}
}
if (correctPosition !== codeLength) {
console.log(`Sorry, you didn't guess the secret code. It was ${secretCode.join(", ")}.`);
}
}
mastermind();
Hi there! Want to support my work?
Now that we've explored the Python and JavaScript implementations of the Mastermind game in a fun and humorous manner, it's time to put your newfound knowledge to the test! Try running the code, cracking the secret code, and adding your own spin to the game.
By working through this entertaining and in-depth guide, you've gained a deeper understanding of the logic and techniques used in both languages. Keep practicing, challenging yourself, and having a laugh as you continue your coding journey. Happy coding, and may the code-breaking force be with you!