Demystifying Data Structures - An Introductory Guide for Beginners

- Published on
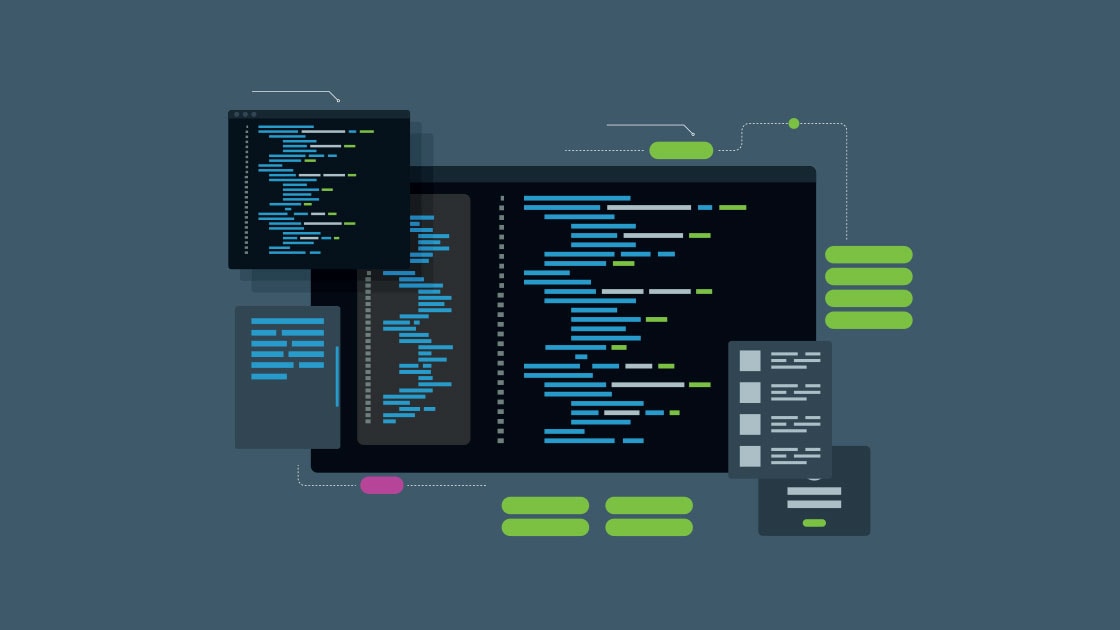
As a programmer, understanding data structures is crucial to optimizing your code and making it efficient. In this article, we'll introduce you to some basic data structures, explain their importance, and provide examples to help you grasp the concepts.
Arrays
An array is a collection of elements, each identified by an index. It's one of the most commonly used data structures, especially when you need to store multiple items of the same data type. In Python, we use lists to represent arrays.
numbers = [1, 2, 3, 4, 5]
print(numbers[1]) # Output: 2 (second element of the list)
Linked Lists
A linked list is a dynamic data structure where each element, known as a node, contains a data value and a reference to the next node in the sequence. Linked lists are useful when you need a flexible data structure with fast insertion and deletion times.
class Node:
def __init__(self, data):
self.data = data
self.next = None
first = Node(1)
second = Node(2)
first.next = second # Connect the first node to the second node
Stacks
A stack is a data structure that follows the Last-In-First-Out (LIFO) principle. It means that the last element added to the stack will be the first one to be removed. Stacks are useful for managing tasks with a specific order, like managing function calls in a programming language.
stack = []
stack.append(1)
stack.append(2)
stack.append(3)
stack.pop() # Removes 3 (the last element added)
Queues
A queue is a data structure that follows the First-In-First-Out (FIFO) principle. It means that the first element added to the queue will be the first one to be removed. Queues are helpful when managing tasks that need to be executed in the order they were added, such as print jobs in a printer.
from collections import deque
queue = deque([1, 2, 3])
queue.append(4)
queue.popleft() # Removes 1 (the first element added)
Hash Tables
A hash table is a data structure that stores key-value pairs and provides fast retrieval based on the key. It uses a hash function to map the keys to specific indices in the table. In Python, dictionaries represent hash tables.
hash_table = {"One": 1, "Two": 2, "Three": 3}
value = hash_table["Two"] # Retrieves the value 2
Mastering data structures will greatly improve your problem-solving skills and make you a more proficient coder. Start exploring these data structures and put them into practice to level up your coding game!