Explore multiple approaches to the FizzBuzz coding challenge, tailored for absolute beginners

- Published on
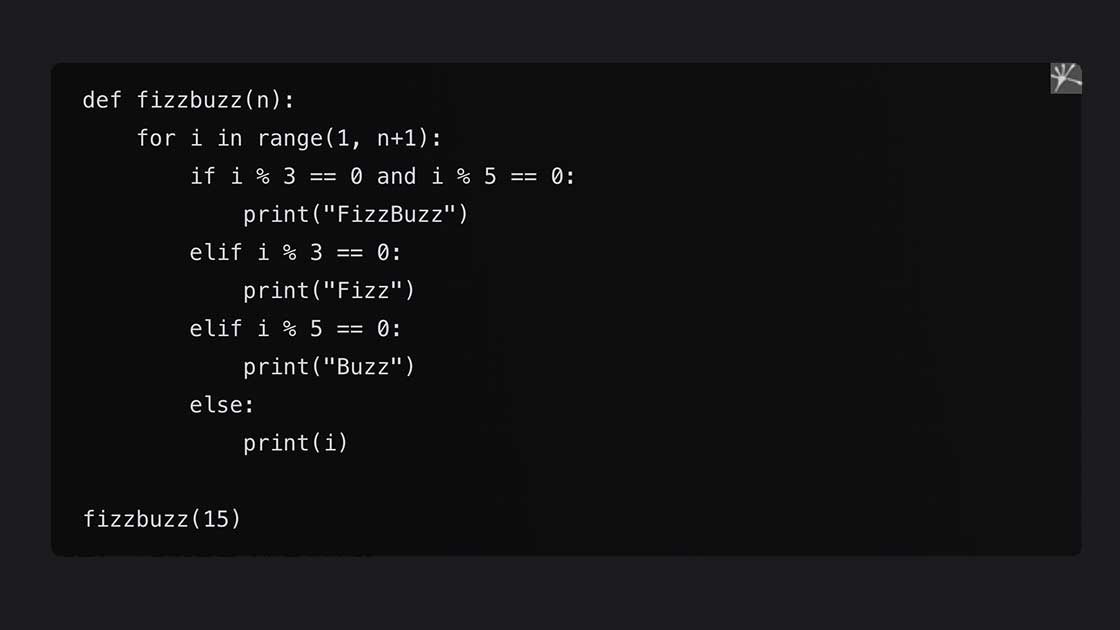
FizzBuzz is a popular coding challenge often used in coding interviews and as an introductory exercise for beginners. The problem is simple, yet it showcases basic programming concepts like loops, conditionals, and modularity. In this article, we'll explore multiple approaches to solve the FizzBuzz problem, discuss their pros and cons, and provide explanations that are easy to understand for absolute beginners.
Problem Statement
Write a program that prints the numbers from 1 to n. For multiples of 3, print "Fizz" instead of the number, and for multiples of 5, print "Buzz". For numbers which are multiples of both 3 and 5, print "FizzBuzz".
Basic Approach
The basic approach uses a for loop and if-else statements to check for multiples of 3 and 5.
def fizzbuzz(n):
for i in range(1, n+1):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
fizzbuzz(15)
Pros:
- Easy to understand and implement
- Familiarizes beginners with loops and conditionals
Cons:
- Not the most concise or efficient approach
String Concatenation Approach
This approach avoids multiple conditional checks by concatenating the "Fizz" and "Buzz" strings based on the number's divisibility.
def fizzbuzz(n):
for i in range(1, n+1):
output = ''
if i % 3 == 0:
output += "Fizz"
if i % 5 == 0:
output += "Buzz"
print(output or i)
fizzbuzz(15)
Pros:
- More concise than the basic approach
- Introduces beginners to string concatenation and truthiness
Cons:
- Slightly more complex for beginners to grasp initially
List Comprehension Approach
This approach leverages list comprehensions, a powerful and concise way to create lists in Python.
def fizzbuzz(n):
result = [
"Fizz" * (i % 3 == 0) + "Buzz" * (i % 5 == 0) or i
for i in range(1, n+1)
]
for item in result:
print(item)
fizzbuzz(15)
Pros:
- Introduces beginners to list comprehensions
- Very concise solution
Cons:
- May be difficult for beginners to understand at first
Using Functions and Modularity
This approach breaks down the problem into smaller, reusable functions.
def fizzbuzz_check(i):
if i % 3 == 0 and i % 5 == 0:
return "FizzBuzz"
elif i % 3 == 0:
return "Fizz"
elif i % 5 == 0:
return "Buzz"
else:
return i
def fizzbuzz(n):
for i in range(1, n+1):
print(fizzbuzz_check(i))
fizzbuzz(15)
Pros:
- Encourages good programming practices like modularity and code reusability
- Easy to understand and maintain
Cons:
- Slightly more verbose than other solutions
Hi there! Want to support my work?
FizzBuzz is an excellent coding problem for beginners to learn basic programming concepts and experiment with different approaches. By trying out these multiple